Project: Task Management System
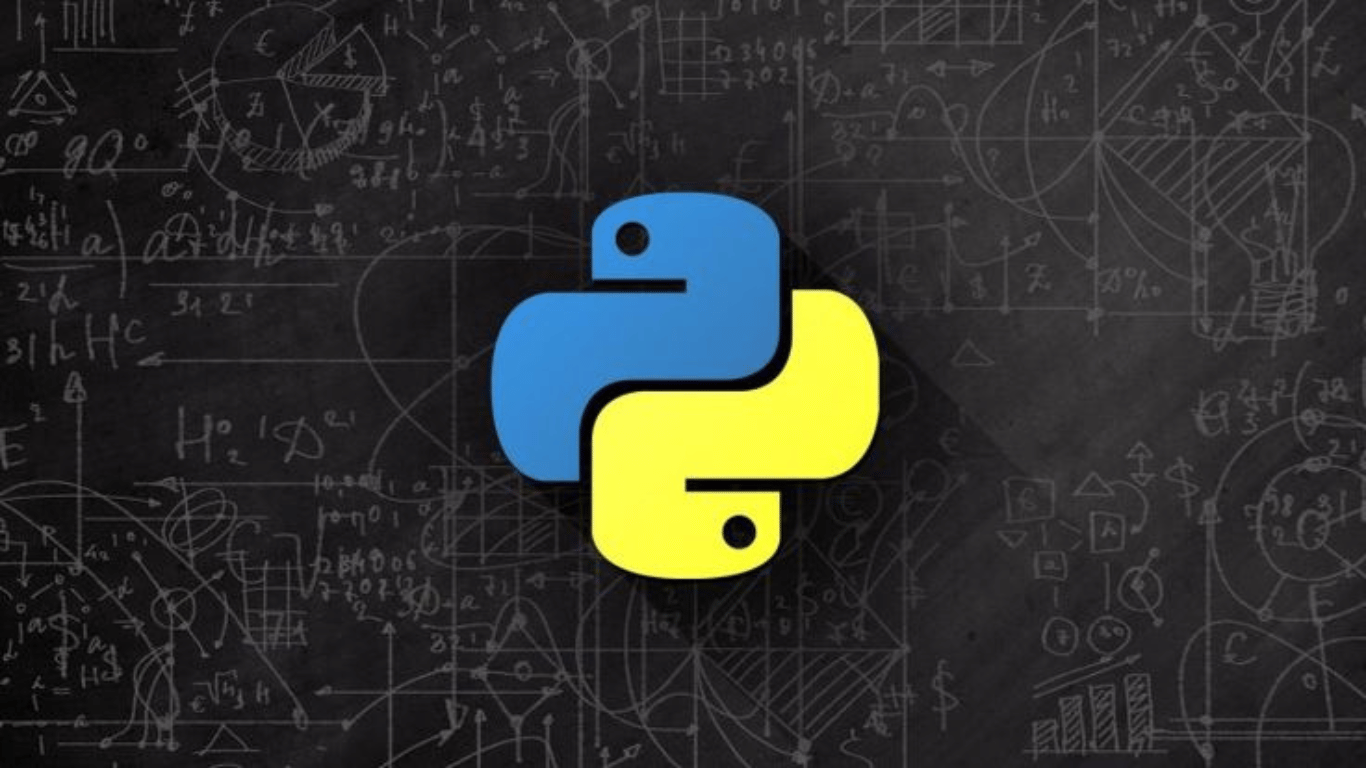
📘 Project Description
A Task Management System allows users to create, update, delete, and view tasks. This system is useful for individuals or teams to manage their daily, weekly, or long-term tasks. Each task will have a title, description, due date, priority level, and status (pending, completed, or overdue).
In this project, you are to create a Python-based task management system where users can interact with tasks in the following ways:
- Add a new task with all the relevant details (title, description, due date, priority, and status).
- Update the details of an existing task, such as changing its status or due date.
- Delete tasks that are no longer relevant or completed.
- View all tasks, filtering by their status or due date.
- Track overdue tasks and notify the user of tasks that need urgent attention.
The system should be able to store the tasks persistently in a JSON file to simulate a database and handle basic operations through a CLI (Command Line Interface).
✅ Main Objectives
- Use classes to model tasks and manage them.
- Implement basic CRUD (Create, Read, Update, Delete) operations for tasks.
- Implement filtering of tasks by status (completed, pending, overdue).
- Persist tasks using a JSON file to store the data.
💻 Solution :
import json
import os
from datetime import datetime
# Task class to represent individual tasks
class Task:
def __init__(self, title, description, due_date, priority, status="pending"):
self.title = title
self.description = description
self.due_date = datetime.strptime(due_date, "%Y-%m-%d")
self.priority = priority
self.status = status
def update(self, title=None, description=None, due_date=None, priority=None, status=None):
if title:
self.title = title
if description:
self.description = description
if due_date:
self.due_date = datetime.strptime(due_date, "%Y-%m-%d")
if priority:
self.priority = priority
if status:
self.status = status
def is_overdue(self):
return self.status != "completed" and datetime.now() > self.due_date
def to_dict(self):
return {
"title": self.title,
"description": self.description,
"due_date": self.due_date.strftime("%Y-%m-%d"),
"priority": self.priority,
"status": self.status
}
@staticmethod
def from_dict(data):
return Task(data['title'], data['description'], data['due_date'], data['priority'], data['status'])
# TaskManager class to handle task operations
class TaskManager:
def __init__(self, filename="tasks.json"):
self.tasks = []
self.filename = filename
self.load()
def add_task(self, task):
self.tasks.append(task)
self.save()
def remove_task(self, title):
self.tasks = [task for task in self.tasks if task.title != title]
self.save()
def update_task(self, title, **kwargs):
task = self.find_task(title)
if task:
task.update(**kwargs)
self.save()
def find_task(self, title):
return next((task for task in self.tasks if task.title == title), None)
def list_tasks(self, status=None):
for task in self.tasks:
if status and task.status != status:
continue
overdue = " (Overdue)" if task.is_overdue() else ""
print(f"{task.title} - {task.status}{overdue}")
print(f" {task.description}")
print(f" Due: {task.due_date.strftime('%Y-%m-%d')} | Priority: {task.priority}")
print()
def save(self):
with open(self.filename, 'w') as f:
json.dump([task.to_dict() for task in self.tasks], f)
def load(self):
if os.path.exists(self.filename):
with open(self.filename, 'r') as f:
data = json.load(f)
self.tasks = [Task.from_dict(task) for task in data]
# Command Line Interface
def main():
manager = TaskManager()
while True:
print("\n=== Task Management System ===")
print("1. Add Task")
print("2. Remove Task")
print("3. Update Task")
print("4. List Tasks")
print("5. Exit")
choice = input("Choose an option: ")
if choice == "1":
title = input("Title: ")
description = input("Description: ")
due_date = input("Due Date (YYYY-MM-DD): ")
priority = input("Priority (low, medium, high): ")
task = Task(title, description, due_date, priority)
manager.add_task(task)
elif choice == "2":
title = input("Title to remove: ")
manager.remove_task(title)
elif choice == "3":
title = input("Title to update: ")
print("Leave blank for fields you don't want to change.")
new_title = input("New Title: ")
new_description = input("New Description: ")
new_due_date = input("New Due Date (YYYY-MM-DD): ")
new_priority = input("New Priority: ")
new_status = input("New Status: ")
manager.update_task(title,
title=new_title if new_title else None,
description=new_description if new_description else None,
due_date=new_due_date if new_due_date else None,
priority=new_priority if new_priority else None,
status=new_status if new_status else None)
elif choice == "4":
status = input("Filter by status (pending, completed, overdue): ")
manager.list_tasks(status=status if status else None)
elif choice == "5":
print("Goodbye!")
break
else:
print("Invalid option. Try again.")
if __name__ == "__main__":
main()
📖 Concepts Used in Detail
- Classes:
Task
andTaskManager
to model tasks and manage them.Task
: Represents an individual task with details like title, description, due date, priority, and status.TaskManager
: Handles CRUD operations such as adding, removing, updating, and listing tasks.
- JSON Serialization: Used to save and load tasks persistently across program runs. The tasks are saved in a JSON file.
- Date and Time: The
datetime
module is used to manage due dates and check for overdue tasks. - Command-Line Interface (CLI): The user interacts with the program through text-based input options for managing tasks.
This task management system provides basic task handling functionality and allows for filtering tasks by their status or overdue status. It uses object-oriented principles to organize tasks and stores the data in JSON format for simplicity and persistence.
If you have any questions , feel free to contact us at [email protected]