Project – Student Management System :
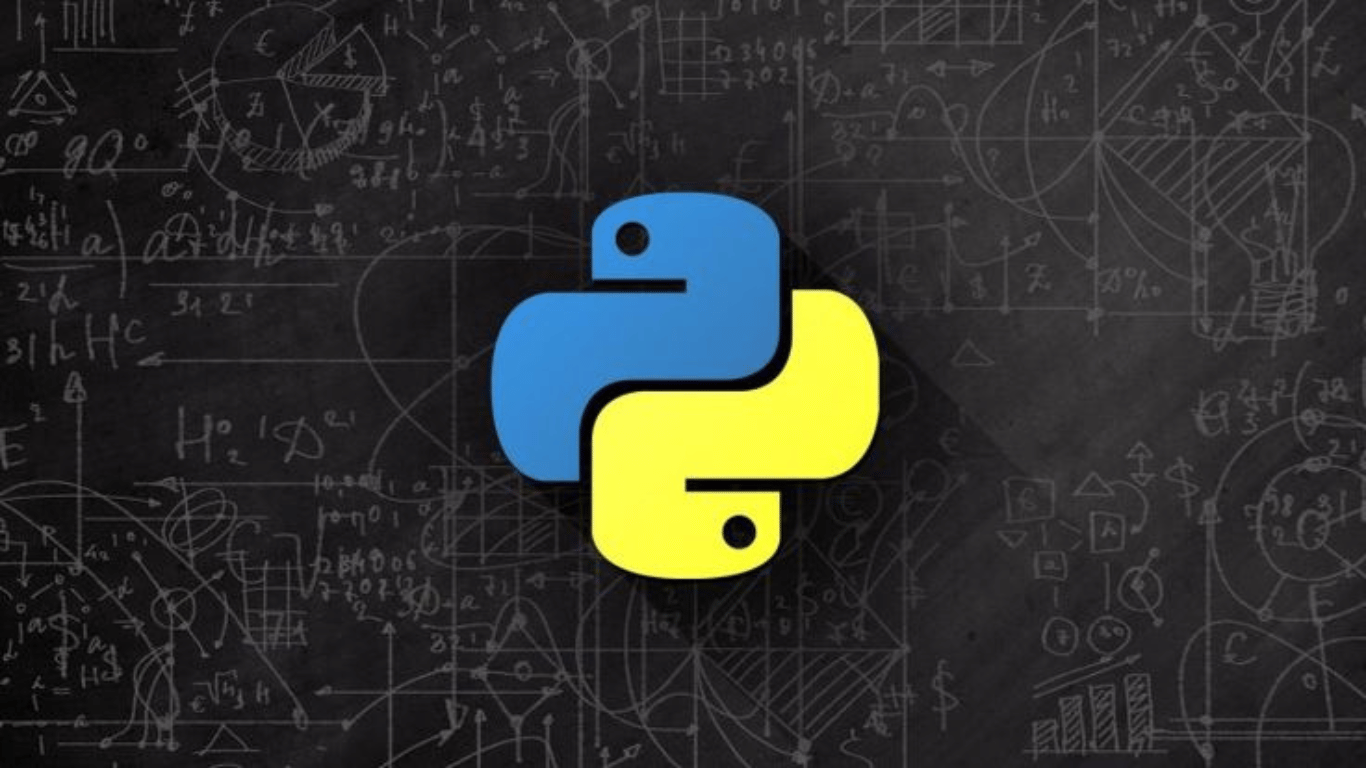
📘 Project Description
In many schools or universities, managing student information is a daily necessity. This includes storing their personal information, grades, attendance, and being able to query this data easily. In this project, you’ll create a Student Management System that allows a school administrator to:
- Add, remove, or update student information
- Track each student’s grades per subject
- Track attendance (present/absent) by date
- Generate grade reports and attendance summaries
- Save and load all data using JSON for persistence
This project will challenge your ability to structure a program using OOP, work with nested data, handle dates, perform basic analysis, and structure clean command-line interaction.
✅ Main Objectives
- Use classes and objects to represent students and the management system
- Implement methods to:
- Add / remove / update student data
- Record and retrieve grades
- Record attendance
- Generate reports:
- Grade averages per student
- Attendance summaries
- Save and load data using JSON
🧠 Concepts Covered
- Object-Oriented Programming
- JSON file I/O
datetime
module- Nested dictionaries/lists
- CLI menu and input validation
💻 Solution :
import json
import os
from datetime import datetime
# Represents a single student
class Student:
def __init__(self, student_id, name):
self.id = student_id
self.name = name
self.grades = {} # subject -> list of grades
self.attendance = {} # date (str) -> "Present" or "Absent"
def add_grade(self, subject, grade):
self.grades.setdefault(subject, []).append(grade)
def mark_attendance(self, date_str, status):
self.attendance[date_str] = status
def average_grade(self, subject=None):
if subject:
grades = self.grades.get(subject, [])
return sum(grades) / len(grades) if grades else 0.0
else:
total, count = 0, 0
for grades in self.grades.values():
total += sum(grades)
count += len(grades)
return total / count if count else 0.0
def attendance_summary(self):
total = len(self.attendance)
present = list(self.attendance.values()).count("Present")
return {"Total Days": total, "Present": present, "Absent": total - present}
def to_dict(self):
return {
"id": self.id,
"name": self.name,
"grades": self.grades,
"attendance": self.attendance
}
@staticmethod
def from_dict(data):
s = Student(data['id'], data['name'])
s.grades = data.get("grades", {})
s.attendance = data.get("attendance", {})
return s
# Manages a list of students
class StudentManager:
def __init__(self, filename="students.json"):
self.filename = filename
self.students = {}
self.load()
def add_student(self, student_id, name):
if student_id in self.students:
print("❌ Student ID already exists.")
return
self.students[student_id] = Student(student_id, name)
self.save()
def remove_student(self, student_id):
if student_id in self.students:
del self.students[student_id]
self.save()
print("✅ Student removed.")
else:
print("❌ Student not found.")
def get_student(self, student_id):
return self.students.get(student_id)
def record_grade(self, student_id, subject, grade):
student = self.get_student(student_id)
if student:
student.add_grade(subject, grade)
self.save()
else:
print("❌ Student not found.")
def record_attendance(self, student_id, date_str, status):
student = self.get_student(student_id)
if student:
student.mark_attendance(date_str, status)
self.save()
else:
print("❌ Student not found.")
def list_students(self):
print("\n📋 Students:")
for s in self.students.values():
print(f"{s.id} - {s.name}")
def save(self):
with open(self.filename, 'w') as f:
json.dump({sid: s.to_dict() for sid, s in self.students.items()}, f)
def load(self):
if not os.path.exists(self.filename):
return
with open(self.filename, 'r') as f:
data = json.load(f)
self.students = {sid: Student.from_dict(sdata) for sid, sdata in data.items()}
# Command Line Interface
def main():
manager = StudentManager()
while True:
print("\n=== Student Management System ===")
print("1. Add Student")
print("2. Remove Student")
print("3. Record Grade")
print("4. Record Attendance")
print("5. Show Grade Report")
print("6. Show Attendance Summary")
print("7. List Students")
print("8. Exit")
choice = input("Choose an option: ")
if choice == "1":
sid = input("Student ID: ")
name = input("Name: ")
manager.add_student(sid, name)
elif choice == "2":
sid = input("Student ID to remove: ")
manager.remove_student(sid)
elif choice == "3":
sid = input("Student ID: ")
subject = input("Subject: ")
try:
grade = float(input("Grade: "))
manager.record_grade(sid, subject, grade)
except ValueError:
print("⚠️ Invalid grade.")
elif choice == "4":
sid = input("Student ID: ")
date = datetime.now().strftime("%Y-%m-%d")
status = input("Attendance (Present/Absent): ")
if status not in ["Present", "Absent"]:
print("⚠️ Must be 'Present' or 'Absent'.")
continue
manager.record_attendance(sid, date, status)
elif choice == "5":
sid = input("Student ID: ")
student = manager.get_student(sid)
if student:
print(f"\n📘 Grade Report for {student.name}")
for subject, grades in student.grades.items():
print(f"{subject}: {grades} (avg: {student.average_grade(subject):.2f})")
print(f"Overall average: {student.average_grade():.2f}")
else:
print("❌ Student not found.")
elif choice == "6":
sid = input("Student ID: ")
student = manager.get_student(sid)
if student:
summary = student.attendance_summary()
print(f"\n🗓️ Attendance for {student.name}")
print(f"Total Days: {summary['Total Days']}")
print(f"Present: {summary['Present']}")
print(f"Absent: {summary['Absent']}")
else:
print("❌ Student not found.")
elif choice == "7":
manager.list_students()
elif choice == "8":
print("👋 Goodbye.")
break
else:
print("❌ Invalid option.")
if __name__ == "__main__":
main()
📖 Concepts Used in Detail
- Classes (
Student
,StudentManager
): For encapsulating student data and operations - JSON Serialization: Save all data in a structured and readable format
datetime
: For automatically getting the current date for attendance- Validation: Ensures safe user input and avoids crashes
- Menu Interface: A clean and looped menu for user interaction
If you have any questions , feel free to contact us at [email protected]