Project – Personal Budget Tracker :
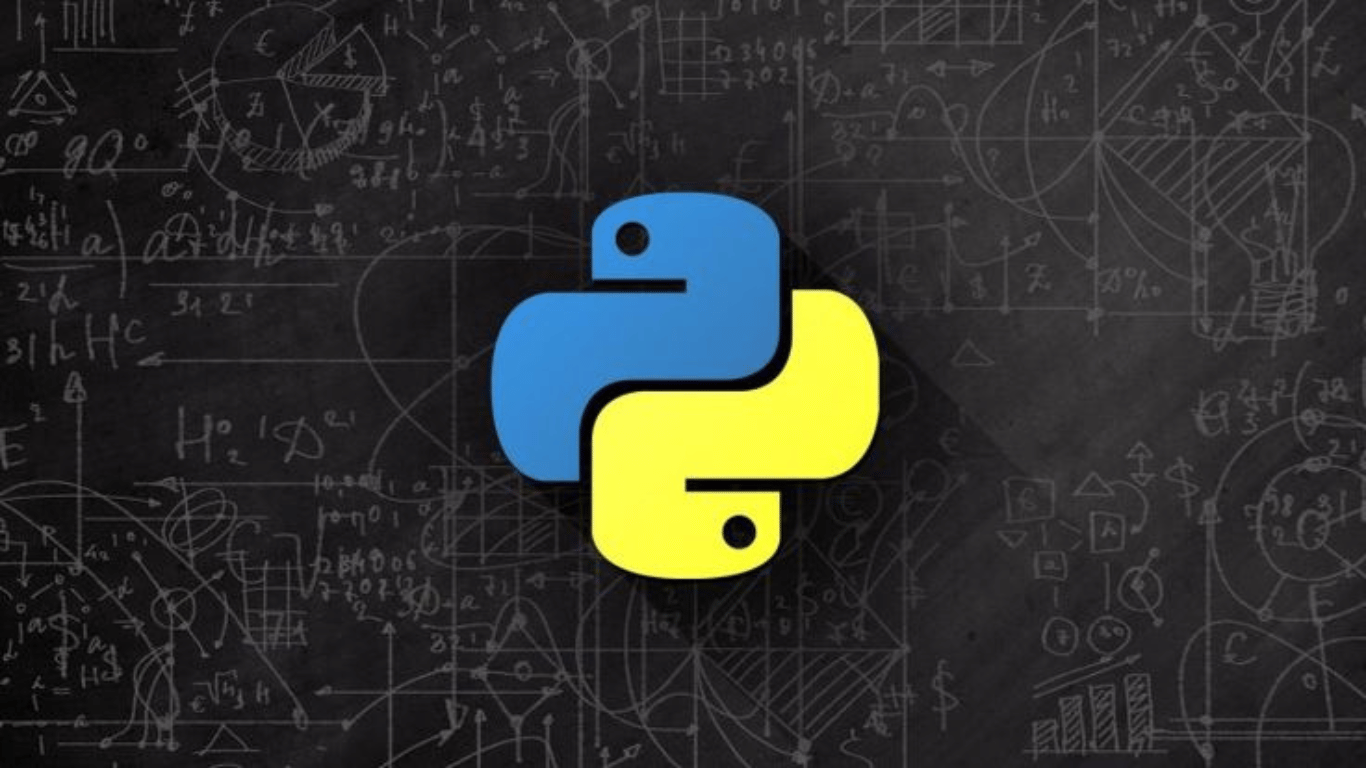
Managing personal finances is an essential life skill. Many people struggle to track their income and expenses, which leads to overspending or missed savings goals. In this project, you’ll build a Personal Budget Tracker in Python that allows users to log their income and expenses, categorize transactions, and get summaries of their financial activity.
Users should be able to:
- Add transactions (income or expense)
- Categorize each transaction (e.g., “Food”, “Rent”, “Salary”)
- Automatically include the current date
- View their total balance
- Get summaries by month and category
- Save and load data using a JSON file so the information persists across sessions
You’ll apply object-oriented programming, basic file I/O, JSON, and input validation. This project will help you learn how to structure real-world applications in Python and prepare your code for scaling or adding features like exporting data or using a graphical interface.
📌 Main Objectives
- Create a class for individual transactions
- Create a class to manage all financial operations
- Add and categorize income and expenses
- Automatically date transactions
- Save all data in a JSON file
- View balance and summaries by month
- Handle invalid inputs with error messages
- Use clean and modular OOP code
✅ Solution :
import json
import os
from datetime import datetime
from collections import defaultdict
# Represents a single income or expense transaction
class Transaction:
def __init__(self, amount, category, t_type, date=None):
self.amount = amount
self.category = category
self.t_type = t_type # 'income' or 'expense'
self.date = date if date else datetime.now().strftime("%Y-%m-%d")
def to_dict(self):
return {
"amount": self.amount,
"category": self.category,
"type": self.t_type,
"date": self.date
}
@staticmethod
def from_dict(data):
return Transaction(
amount=data['amount'],
category=data['category'],
t_type=data['type'],
date=data['date']
)
# Manages the collection of transactions and user operations
class BudgetTracker:
def __init__(self, filename="budget_data.json"):
self.filename = filename
self.transactions = []
self.load_data()
def add_transaction(self, amount, category, t_type):
if t_type not in ["income", "expense"]:
print("❌ Invalid type. Use 'income' or 'expense'.")
return
transaction = Transaction(amount, category, t_type)
self.transactions.append(transaction)
self.save_data()
print(f"✅ Added {t_type} of {amount} in category '{category}'.")
def show_balance(self):
income = sum(t.amount for t in self.transactions if t.t_type == "income")
expenses = sum(t.amount for t in self.transactions if t.t_type == "expense")
print(f"💰 Total Income: {income}")
print(f"💸 Total Expenses: {expenses}")
print(f"📊 Balance: {income - expenses}")
def monthly_summary(self, year_month):
summary = defaultdict(lambda: {"income": 0, "expense": 0})
for t in self.transactions:
if t.date.startswith(year_month):
summary[t.category][t.t_type] += t.amount
print(f"\n📅 Summary for {year_month}:")
for category, totals in summary.items():
print(f"{category}: +{totals['income']} | -{totals['expense']}")
def save_data(self):
with open(self.filename, 'w') as f:
json.dump([t.to_dict() for t in self.transactions], f)
def load_data(self):
if not os.path.exists(self.filename):
return
with open(self.filename, 'r') as f:
data = json.load(f)
self.transactions = [Transaction.from_dict(d) for d in data]
# Simple CLI interface
def main():
tracker = BudgetTracker()
while True:
print("\n=== Budget Tracker Menu ===")
print("1. Add Income")
print("2. Add Expense")
print("3. Show Balance")
print("4. Monthly Summary")
print("5. Exit")
choice = input("Choose an option: ")
if choice == "1":
try:
amount = float(input("Enter amount: "))
category = input("Enter category: ")
tracker.add_transaction(amount, category, "income")
except ValueError:
print("⚠️ Invalid amount.")
elif choice == "2":
try:
amount = float(input("Enter amount: "))
category = input("Enter category: ")
tracker.add_transaction(amount, category, "expense")
except ValueError:
print("⚠️ Invalid amount.")
elif choice == "3":
tracker.show_balance()
elif choice == "4":
year_month = input("Enter year and month (YYYY-MM): ")
tracker.monthly_summary(year_month)
elif choice == "5":
print("👋 Goodbye!")
break
else:
print("❌ Invalid option.")
if __name__ == "__main__":
main()
📖 Concepts and Tools Used
- OOP (Classes): Used to define clear structure (
Transaction
,BudgetTracker
) - JSON: Used to store and load transaction data
- Datetime: To add the current date automatically to each transaction
- defaultdict: For grouped monthly summaries without worrying about missing keys
- Error Handling: Basic try/except and input validation
- File I/O: Reading/writing to a
.json
file for persistent storage
If you have any questions , feel free to contact us at [email protected]