Project – Library Management System:
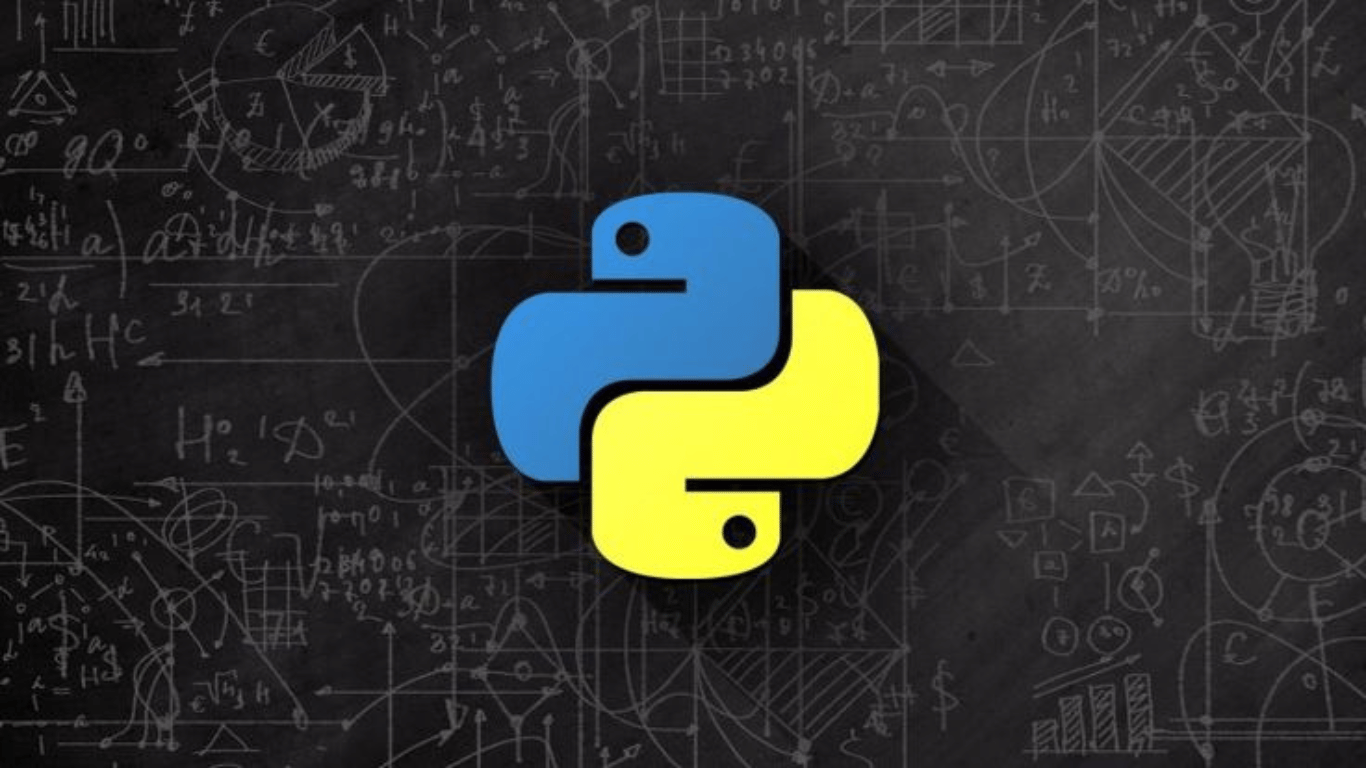
You’re going to build a Library Management System in Python. This system allows a user to:
- Add books to the library.
- Display available books.
- Borrow a book (mark it as borrowed).
- Return a book.
- Save and load library data from a file so it persists between runs.
This project will give you practical experience with:
- Object-Oriented Programming (classes and objects)
- Lists and dictionaries
- File I/O (reading/writing files)
- JSON serialization
- Error handling
- Data persistence
The project will consist of a single Python file and one JSON file (library_data.json
) to store book data.
✅ Solution :
import json
import os
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
self.is_borrowed = False
def to_dict(self):
return {
"title": self.title,
"author": self.author,
"is_borrowed": self.is_borrowed
}
@staticmethod
def from_dict(data):
book = Book(data['title'], data['author'])
book.is_borrowed = data['is_borrowed']
return book
class Library:
def __init__(self, data_file='library_data.json'):
self.books = []
self.data_file = data_file
self.load_books()
def add_book(self, title, author):
self.books.append(Book(title, author))
self.save_books()
print(f"✅ Book '{title}' added to the library.")
def display_books(self):
if not self.books:
print("📭 No books in the library.")
return
print("\n📚 Available books:")
for idx, book in enumerate(self.books):
status = "Borrowed" if book.is_borrowed else "Available"
print(f"{idx+1}. {book.title} by {book.author} - [{status}]")
def borrow_book(self, index):
try:
book = self.books[index]
if book.is_borrowed:
print("⚠️ This book is already borrowed.")
else:
book.is_borrowed = True
self.save_books()
print(f"📖 You have borrowed '{book.title}'.")
except IndexError:
print("⚠️ Invalid book number.")
def return_book(self, index):
try:
book = self.books[index]
if not book.is_borrowed:
print("⚠️ This book was not borrowed.")
else:
book.is_borrowed = False
self.save_books()
print(f"🔁 You have returned '{book.title}'.")
except IndexError:
print("⚠️ Invalid book number.")
def save_books(self):
data = [book.to_dict() for book in self.books]
with open(self.data_file, 'w') as f:
json.dump(data, f)
def load_books(self):
if not os.path.exists(self.data_file):
return
with open(self.data_file, 'r') as f:
data = json.load(f)
self.books = [Book.from_dict(b) for b in data]
def main():
library = Library()
while True:
print("\n📌 Library Menu")
print("1. Add Book")
print("2. Show Books")
print("3. Borrow Book")
print("4. Return Book")
print("5. Exit")
choice = input("Enter choice: ")
if choice == "1":
title = input("Enter book title: ")
author = input("Enter author name: ")
library.add_book(title, author)
elif choice == "2":
library.display_books()
elif choice == "3":
library.display_books()
try:
index = int(input("Enter book number to borrow: ")) - 1
library.borrow_book(index)
except ValueError:
print("⚠️ Please enter a valid number.")
elif choice == "4":
library.display_books()
try:
index = int(input("Enter book number to return: ")) - 1
library.return_book(index)
except ValueError:
print("⚠️ Please enter a valid number.")
elif choice == "5":
print("👋 Goodbye!")
break
else:
print("❌ Invalid choice. Try again.")
if __name__ == "__main__":
main()
🧠 Detailed Explanation of Key Concepts
📦 Book
Class
- Represents a book with a title, author, and borrowed status.
to_dict()
: Converts the book into a dictionary for saving.from_dict()
: Re-creates aBook
from a dictionary when loading from file.
🏛️ Library
Class
- Stores a list of books and manages saving/loading from a JSON file.
add_book()
: Adds a new book to the list and saves.display_books()
: Prints all books with their status.borrow_book(index)
: Changesis_borrowed
toTrue
.return_book(index)
: Changesis_borrowed
toFalse
.save_books()
: Serializes the list into a JSON file.load_books()
: Loads books from file if it exists.
📁 File Handling
- Uses
json
to serialize and deserialize book data. - Book data is saved in
library_data.json
, allowing persistent storage between runs.
🔁 Main Loop
- Displays a menu and processes user input.
- Handles invalid input and guides the user through the process.
✅ Sample Run (User View)
📌 Library Menu
1. Add Book
2. Show Books
3. Borrow Book
4. Return Book
5. Exit
Enter choice: 1
Enter book title: 1984
Enter author name: George Orwell
✅ Book '1984' added to the library.
📌 Library Menu
2. Show Books
📚 Available books:
1. 1984 by George Orwell - [Available]
🧩 Ideas for Extensions
If you want to make this project more complex:
- Add user accounts with login/signup.
- Track which user borrowed which book.
- Add due dates and late return penalties.
- Create a GUI version using Tkinter or PyQt.
If you have any questions , feel free to contact us at [email protected] .