Project – 📝 To-Do List Application :
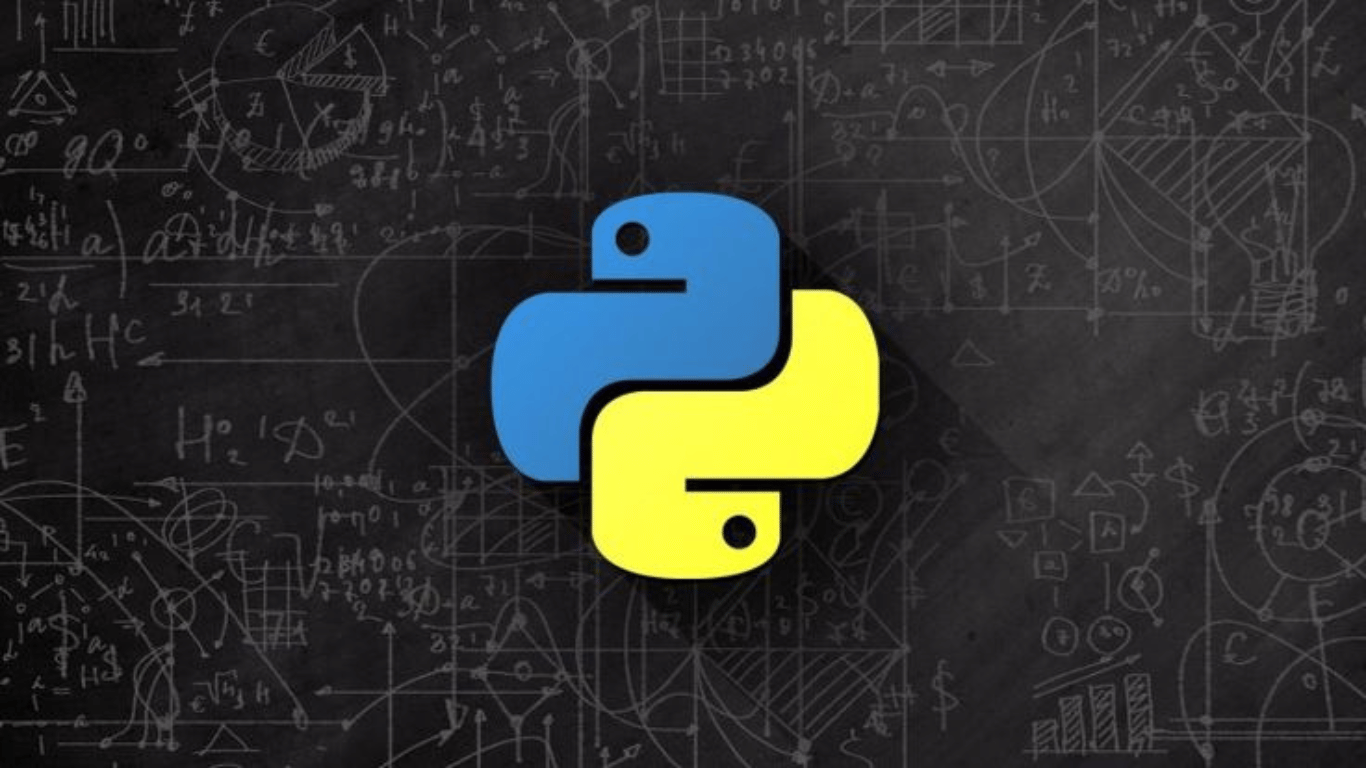
📌 Objective:
Create a simple command-line To-Do list manager in Python. This mini project will help you understand the use of:
- Lists and dictionaries
- Functions and control flow (
if
,else
,while
) - User input handling
- Basic error checking (
try/except
) - Console-based user interaction
📋 Features to implement:
- Add a new task
The user should be able to enter a task name. The task should be added to a list with a default status of not completed. - View all tasks
Display all the tasks with a number and their status (✅ completed / ❌ not completed). - Mark a task as completed
The user can enter the number of the task they wish to mark as completed. - Exit the application
Gracefully terminate the program when the user chooses to exit.
Each task will be represented as a dictionary with two keys:
"name"
: the task name"done"
: a boolean indicating if the task is completed
This project encourages clean user interaction through the console and teaches how to maintain a list of structured items in memory.
✅ Solution :
# Simple Console To-Do List App
tasks = [] # List to hold all task dictionaries
while True:
print("\n1. Add | 2. View | 3. Complete | 4. Exit")
choice = input("Choose an option: ")
if choice == "1":
name = input("Enter task name: ")
tasks.append({"name": name, "done": False}) # Add task as not done
elif choice == "2":
if not tasks:
print("📭 No tasks yet.")
for i, task in enumerate(tasks):
status = "✔" if task["done"] else "❌"
print(f"{i+1}. {task['name']} [{status}]") # Show task with status
elif choice == "3":
try:
number = int(input("Enter task number to mark as complete: ")) - 1
if 0 <= number < len(tasks):
tasks[number]["done"] = True
print("✅ Task marked as complete.")
else:
print("⚠️ Invalid task number.")
except ValueError:
print("⚠️ Please enter a valid number.")
elif choice == "4":
print("👋 Exiting... Goodbye!")
break # Exit the loop and program
else:
print("❌ Invalid choice. Please try again.")
🧠 Explanation of the Code Components
Code Element | Purpose |
---|---|
tasks = [] | Initializes an empty list to store tasks. |
{"name": ..., "done": False} | Represents each task as a dictionary with a name and a done status. |
while True: | Keeps the program running until the user chooses to exit. |
input() | Gets user input for menu choices or task names/numbers. |
enumerate(tasks) | Loops through tasks with an index so we can number them for the user. |
if choice == ... | Handles each menu option based on user input. |
try/except | Prevents crashes when users enter invalid input for task numbers. |
break | Ends the loop when the user chooses to exit. |
For any questions , please feel free to contact us at [email protected]