AI-Powered Habit Tracker
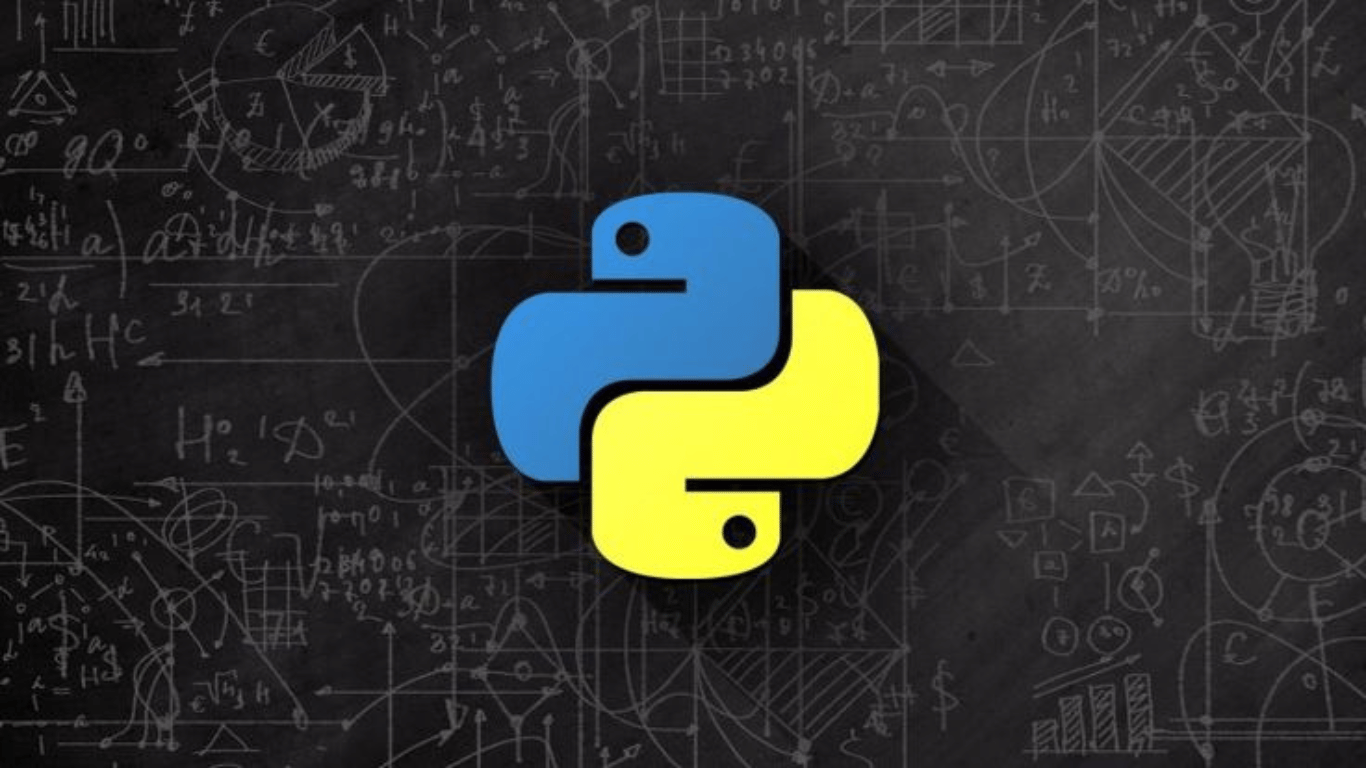
The AI-Powered Habit Tracker is a console-based Python application that helps users build and maintain good habits. The system allows users to register habits, log daily progress, and visualize their streaks over time using ASCII-style charts. Additionally, the application uses a basic machine learning model (via scikit-learn) to predict how likely a user is to complete a habit based on past performance and time of day.
Functionalities:
- Habit Registration
- Users can create new habits (e.g., “Drink Water”, “Read Book”)
- Each habit has a goal time (e.g., 8 PM) and category (e.g., Health, Learning)
- Daily Tracking
- Users can mark a habit as complete for a specific day
- Automatic timestamping
- Streak Visualization
- Displays current and longest streaks
- ASCII bar chart for last 7 days per habit
- Prediction System
- Based on past logs, it estimates the likelihood of completion using logistic regression
- Input features: time of day, past success rate
Solution :
Habit Class :
import datetime
import pickle
from collections import defaultdict
from sklearn.linear_model import LogisticRegression
# ---------------------------
# Habit class: stores data and history
# ---------------------------
class Habit:
def __init__(self, name, category, goal_hour):
self.name = name
self.category = category
self.goal_hour = goal_hour
self.history = {} # key = date string, value = 1 if completed
def mark_done(self, date=None):
date = date or datetime.date.today().isoformat()
self.history[date] = 1
def streaks(self):
dates = sorted(self.history.keys())
current_streak = longest_streak = 0
prev_date = None
for date_str in dates:
date = datetime.date.fromisoformat(date_str)
if prev_date and (date - prev_date).days == 1:
current_streak += 1
else:
current_streak = 1
longest_streak = max(longest_streak, current_streak)
prev_date = date
return current_streak, longest_streak
def display_chart(self):
print(f"== {self.name} (Last 7 Days) ==")
today = datetime.date.today()
for i in range(6, -1, -1):
day = today - datetime.timedelta(days=i)
mark = '✔' if day.isoformat() in self.history else '✘'
print(f"{day.strftime('%a')} | {mark}")
print()
HabitTracker :
# ---------------------------
# HabitTracker: Manages all habits and ML model
# ---------------------------
class HabitTracker:
def __init__(self):
self.habits = {}
self.ml_model = LogisticRegression()
self.ml_data = []
def add_habit(self, name, category, hour):
self.habits[name] = Habit(name, category, hour)
def mark_habit(self, name, time=None):
habit = self.habits.get(name)
if not habit:
print("Habit not found.")
return
habit.mark_done()
time = time or datetime.datetime.now().hour
self.ml_data.append(([time], 1)) # label = 1 (completed)
print(f"{name} marked as done.")
def train_model(self):
if len(self.ml_data) < 5:
print("Not enough data to train.")
return
X, y = zip(*self.ml_data)
self.ml_model.fit(X, y)
print("Prediction model trained.")
def predict_habit(self, name, time):
habit = self.habits.get(name)
if not habit:
print("Habit not found.")
return
if not hasattr(self.ml_model, "coef_"):
print("Model not trained.")
return
prob = self.ml_model.predict_proba([[time]])[0][1]
print(f"Likelihood of completing '{name}' at {time}:00 is {prob:.2f}")
def summary(self):
for habit in self.habits.values():
streak, max_streak = habit.streaks()
print(f"{habit.name} | Current: {streak} | Longest: {max_streak}")
habit.display_chart()
Example Usage :
# ---------------------------
# Example Usage
# ---------------------------
if __name__ == "__main__":
tracker = HabitTracker()
tracker.add_habit("Read Book", "Learning", 21)
tracker.add_habit("Exercise", "Health", 7)
tracker.mark_habit("Read Book")
tracker.mark_habit("Exercise", time=7)
tracker.train_model()
tracker.predict_habit("Exercise", time=7)
tracker.summary()
Explanation of the Logic
This Python program simulates a habit tracking system enhanced with basic AI prediction. The user interacts with a console to register habits, mark them daily, and receive predictions on their future behavior. The app follows an OOP approach with two primary classes:
1. Habit Class
Each Habit stores the name, category, goal time, and a history dictionary. When mark_done()
is called, it logs the current date. The streaks()
method calculates:
- Current streak: how many days in a row the habit has been completed
- Longest streak: the max continuous completion period
It also contains a display_chart()
method that renders an ASCII chart of the past 7 days using ✔ or ✘.
2. HabitTracker Class
The HabitTracker class manages:
- A dictionary of habits
- A list of training data for machine learning
- A
LogisticRegression
model fromscikit-learn
Each time a habit is marked, the system adds training data with the hour and a completion label. When enough samples exist, train_model()
creates a simple logistic regression classifier. The method predict_habit()
estimates the probability of completing a habit at a certain hour using predict_proba()
.
Key Python Concepts Used
Concept | Description |
---|---|
class | Used to define Habit and HabitTracker objects |
datetime | Tracks current date and time for logging |
defaultdict | (from collections) could help in extending streak logic |
scikit-learn | Used to fit a basic logistic regression model for predictions |
dict | Stores habit history and lookup via names |
list of tuples | Training dataset for ML (([hour], label) ) |
if __name__ == "__main__" | Ensures main logic only runs when the script is directly executed |
If you have any questions , feel free to contact us at [email protected]