Project: Simple Text-Based Adventure Game
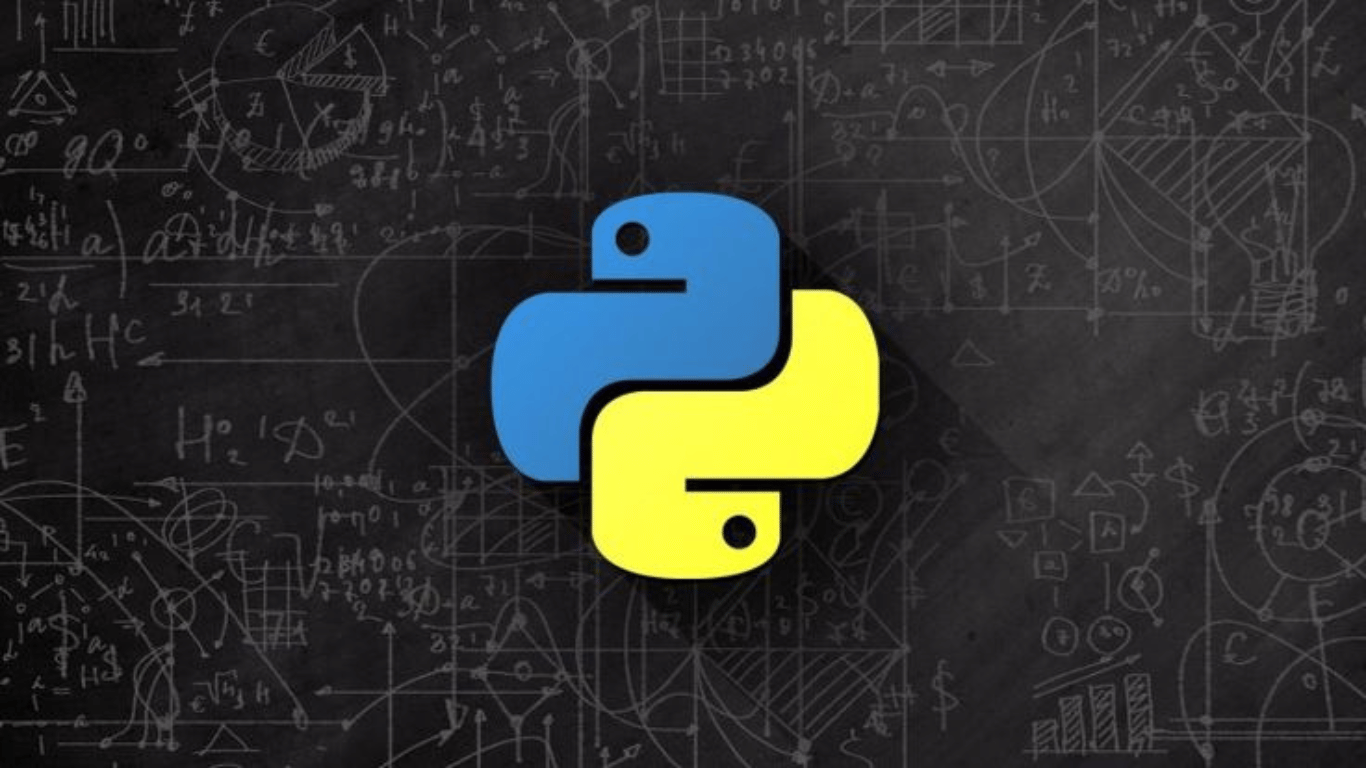
📘 Project Description
In this project, you will create a Text-Based Adventure Game where the player navigates through different locations, interacts with objects, and makes choices that affect the outcome of the game. The player will move between different rooms, solve simple puzzles, and collect items to progress in the game. The game will have a limited set of actions, such as moving to another room, picking up items, and using items to solve challenges.
The goal is to create an interactive game loop where the player’s choices lead to different outcomes. The player should also have the opportunity to “win” or “lose” the game based on the actions taken throughout the adventure.
🎯 What you need to implement:
- Room Class: Each room will have a description, a list of items in the room, and a set of possible directions the player can move.
- Player Class: The player will have a list of items they’ve collected, their current room, and their health (if applicable).
- Game Loop: A loop that prompts the player for actions, processes the player’s input, and updates the state of the game.
- Items: Implement items that the player can pick up and use (e.g., keys, health potions, etc.).
- Multiple Outcomes: Depending on the player’s choices, the game may lead to different endings (success or failure).
💻 Python Code Solution
import random
class Item:
def __init__(self, name, description):
self.name = name
self.description = description
def __repr__(self):
return f"{self.name}: {self.description}"
class Room:
def __init__(self, name, description, items=None, directions=None):
self.name = name
self.description = description
self.items = items if items else []
self.directions = directions if directions else {}
def describe(self):
print(self.description)
print("Items in the room:", ", ".join([item.name for item in self.items]))
print("Exits:", ", ".join(self.directions.keys()))
class Player:
def __init__(self, name):
self.name = name
self.inventory = []
self.current_room = None
def pick_up(self, item):
self.inventory.append(item)
self.current_room.items.remove(item)
print(f"You picked up: {item.name}")
def use_item(self, item_name):
item = next((item for item in self.inventory if item.name.lower() == item_name.lower()), None)
if item:
print(f"You used {item.name}. {item.description}")
self.inventory.remove(item)
else:
print(f"You don't have a {item_name}.")
def move(self, direction):
if direction in self.current_room.directions:
self.current_room = self.current_room.directions[direction]
print(f"You moved to {self.current_room.name}.")
else:
print("You can't go that way.")
class Game:
def __init__(self):
self.rooms = self.create_rooms()
self.player = Player(name="Adventurer")
self.player.current_room = self.rooms["start"]
def create_rooms(self):
room1 = Room("Start Room", "You are in a small room with a door leading to the north.",
items=[Item("Torch", "A wooden torch that might come in handy.")],
directions={"north": "forest"})
room2 = Room("Forest", "You are in a dense forest, the trees are tall, and you hear birds chirping.",
items=[Item("Key", "A rusty key with an unknown purpose.")],
directions={"south": room1, "east": "cave"})
room3 = Room("Cave", "It's dark inside the cave, and you can hear water dripping.",
items=[Item("Health Potion", "A small vial of red liquid.")],
directions={"west": room2})
return {"start": room1, "forest": room2, "cave": room3}
def start(self):
print("Welcome to the Adventure Game!")
while True:
self.player.current_room.describe()
action = input("What would you like to do? (move, pick up, use, quit): ").strip().lower()
if action == "quit":
print("Thanks for playing!")
break
elif action == "move":
direction = input("Which direction? (north, south, east, west): ").strip().lower()
self.player.move(direction)
elif action == "pick up":
item_name = input("What item would you like to pick up?: ").strip()
item = next((item for item in self.player.current_room.items if item.name.lower() == item_name.lower()), None)
if item:
self.player.pick_up(item)
else:
print(f"{item_name} is not in the room.")
elif action == "use":
item_name = input("Which item would you like to use?: ").strip()
self.player.use_item(item_name)
else:
print("Invalid action. Please try again.")
print("\n---")
if __name__ == "__main__":
game = Game()
game.start()
📝 Detailed Explanation of the Solution
1. Item Class
- Attributes:
name
: The name of the item (e.g., “Torch”, “Key”).description
: A brief description of the item’s use (e.g., “A wooden torch that might come in handy”).
- Methods:
__repr__
: This method defines how the item will be represented when printed, showing its name and description.
2. Room Class
- Attributes:
name
: The name of the room (e.g., “Start Room”).description
: A description of the room’s appearance and features.items
: A list of items in the room that the player can interact with.directions
: A dictionary that maps directions (north, south, etc.) to other rooms or places.
- Methods:
describe
: This method prints the description of the room, lists the items in the room, and shows the available exits.
3. Player Class
- Attributes:
name
: The name of the player.inventory
: A list of items the player has collected.current_room
: The room the player is currently in.
- Methods:
pick_up
: Adds an item to the player’s inventory and removes it from the current room.use_item
: Allows the player to use an item from their inventory.move
: Allows the player to move to a different room by specifying a direction.
4. Game Class
- Attributes:
rooms
: A dictionary of rooms in the game (the player can move between these).player
: An instance of thePlayer
class that represents the player.
- Methods:
create_rooms
: This method creates the rooms, assigns directions, and adds items to each room.start
: This method starts the game loop, asking the player for actions (move, pick up, use, or quit) and updating the game state.
5. Game Loop
- The game runs inside an infinite loop, where the player interacts with the game by entering commands. The loop continues until the player decides to quit.
🧠 Key Concepts Used:
- Object-Oriented Programming (OOP): The game is built using OOP principles where the
Item
,Room
,Player
, andGame
classes represent the different components of the game world. - Game Loop: The game continually prompts the player for actions and updates based on the player’s input, simulating an interactive experience.
- Interaction and State Changes: The player can affect the game world (by picking up items, moving rooms, etc.), and the game state changes accordingly.
⚡ Potential Enhancements:
- Inventory Limit: Add a maximum number of items the player can carry.
- Randomized Events: Introduce random events or encounters (e.g., monsters, hidden rooms).
- Multiple Endings: Based on player choices, different game outcomes could be triggered (e.g., finding the treasure or getting trapped).
- Graphics: Move from a text-based game to a graphical user interface (GUI) using libraries like
tkinter
orpygame
.
This project teaches basic game design and the use of object-oriented programming to model a simple interactive world.